Pythonscripts für die Verarbeitungswerkzeuge schreiben (QGIS3)¶
Man kann eigenständige pygis Skripts schreiben und sie mit der Python Konsole in QGIS ausführen. Mit etwas Anpassung kann man diese Skripts auch innerhalb der Verarbeitungswerkzeuge verwenden. Das hat einige Vorteile. Die Benutzereingabe und die Ausgabe von Ergebnissen ist viel einfacher, da die Verarbeitungswerkzeuge eine Benutzerschnittstelle dafür vorsehen. Skripte in den Verarbeitungswerkzeugen können außerdem von jedem anderen Verarbeitungswerkzeug verwendet werden und sie können mit der Stapelverarbeitung mehrere Eingabedateien verarbeiten. Diese Anleitung zeigt, wie man ein Pythonskript schreibt, das dann Teil der Verarbeitungswerkzeuge wird.
Bemerkung
Die Schnittstelle der Verarbeitungswerkzeuge wurde mit QGIS3 komplett überholt. Unter this guide findet man Tips und Hinweise dazu.
Überblick über die Aufgabe¶
Unser Skript wird eine dissolve-Operation basierend auf einem vom Benutzer vorgegebenen Feld ausführen. Es wird außerdem die Werte eines anderen Feldes die zusammengefassten Features aufsummieren. Bei diesem Beispiel werden wir eine shape-Datei der Welt basierend auf dem Attribut CONTINENT
zusammenfassen und die Werte im Attribut POP_EST
aufsummieren, um die Gesamtbevölkerung in der zusammengefassten Region zu bestimmen.
Beschaffung der Daten¶
We will use the Admin 0 - Countries dataset from Natural Earth.
Download the Admin 0 - countries shapefile..
Data Source [NATURALEARTH]
Der Einfachheit halber kannst du den Layer in einem Geopackage direkt unter folgendem link herunterladen:
Arbeitsablauf¶
Gehe im QGIS Browser Bereich zum Verzeichnis in dem die heruntergeladenen Daten abgelegt sind. Erweitere die
zip
oder diegpkg
Datei und wähle den Layerne_10m_admin_0_countries
. Ziehe den Layer in den Arbeitsbereich.
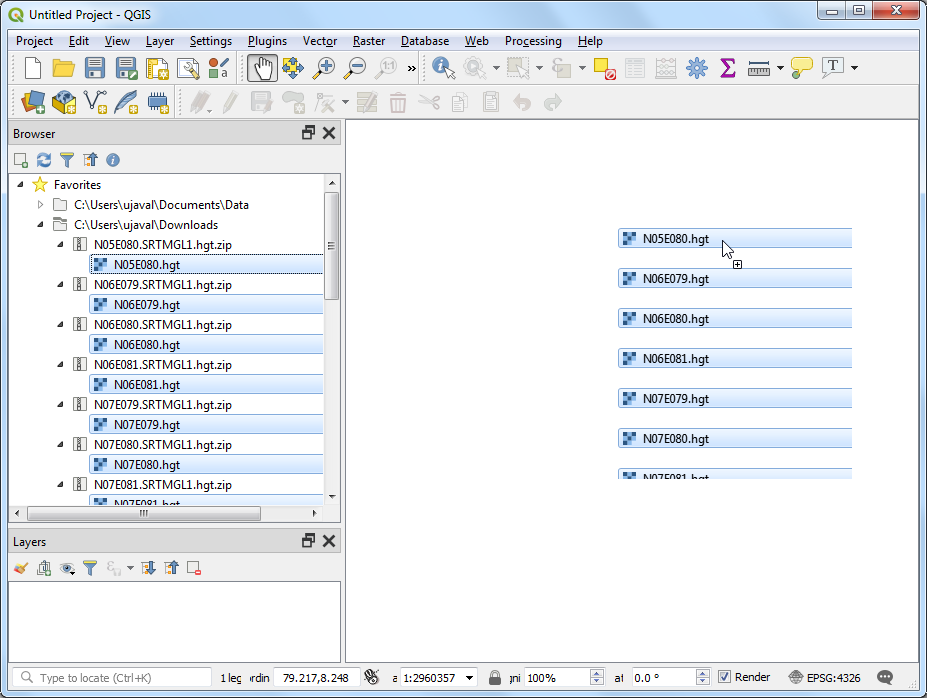
Gehe im Menü zu Skripte in der Toolbar und wähle Neues Skript aus Vorlage erzeugen.
. Klicke auf den Knopf
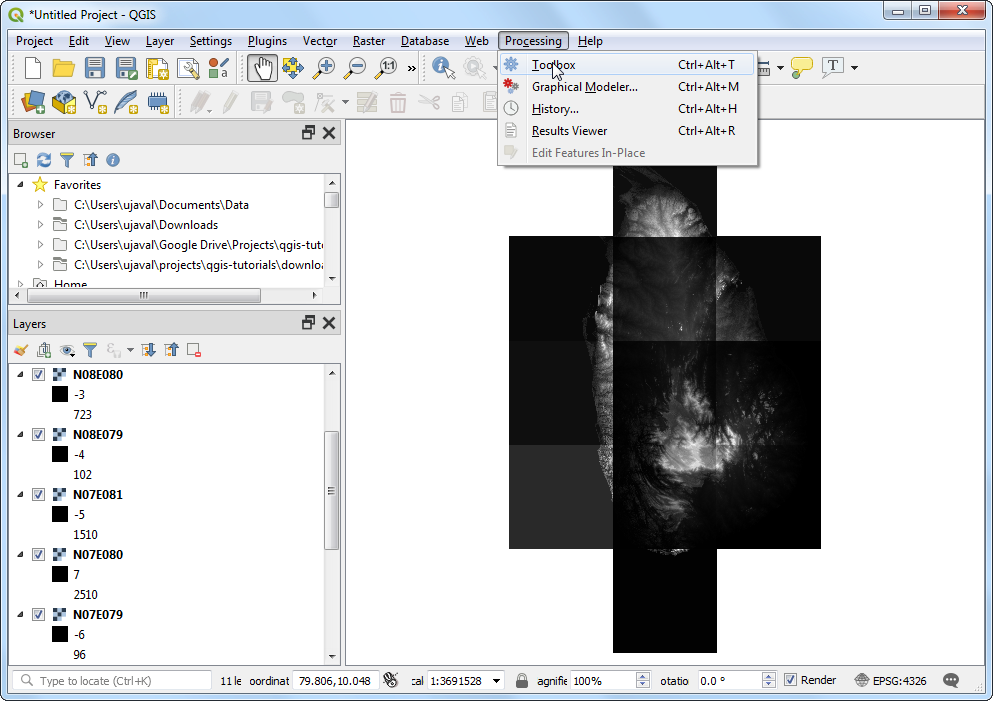
Die Vorlage enthält die Textbausteine, die für das Prozessierungsframework erforderlich sind, um das Skript als Prozessierungsskript zu erkennen und die Ein- und Ausgabe zu verwalten. Wir werden die Vorlage nun an unsere Anforderungen anpassen. Zuerst ändern wir den Klassennamen von
ExampleProcessingAlgorithm
zuDissolveProcessingAlgorithm
. Dieser Name muss auch unter dercreateInstance
Methode geändert werden. Wir fügen außerdem eine Erläuterung mit einer Beschreibung des Algorithmus ein.
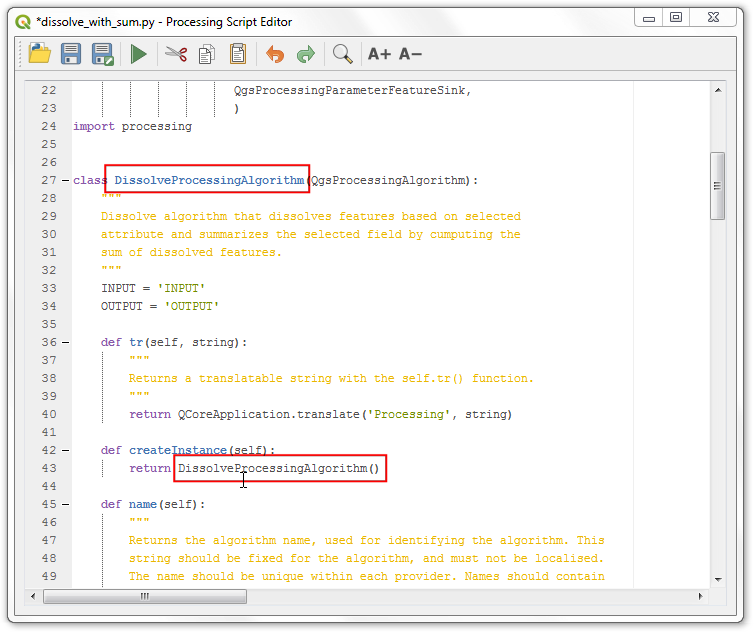
Beim Herunterscrollen sehen wir Methoden, die Namen, Gruppe, Beschreibung usw. im script zuweisen. wir ändern die Rückgabewerte für die name Methode zu
dissolve_with_sum
, für die displayName Methode zuDissolve with Sum
, für die group Methode und die groupId Methode zuscripts
. Wir ändern den Rückgabewert der shortHelpString Methode zu einer Beschreibung, die dem Nutzer erscheinen wird. Klicke auf den Speichern Knopf.
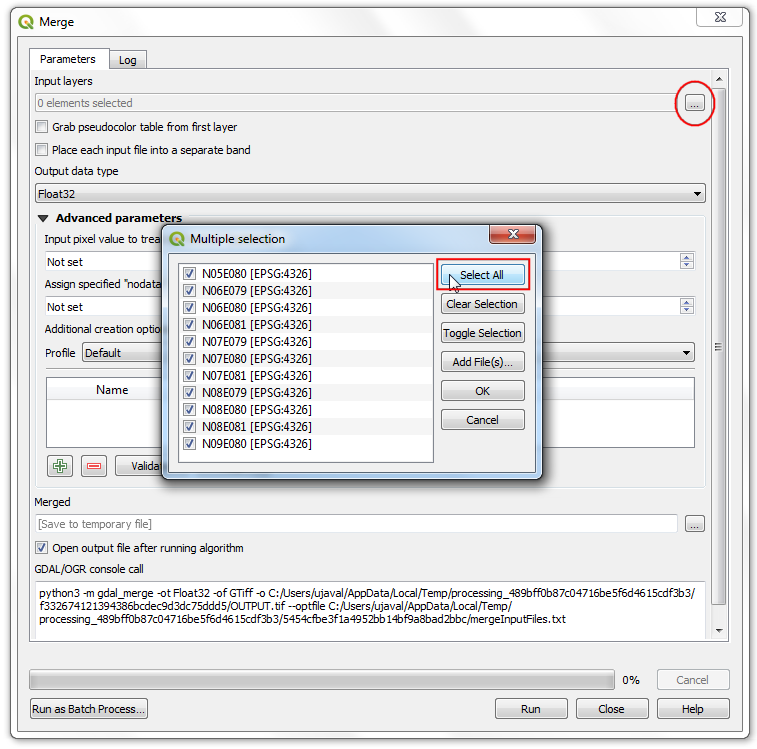
Wir nennen das Skript
dissolve_with_sum
und speichern es im vorgegebenen Verzeichnis .
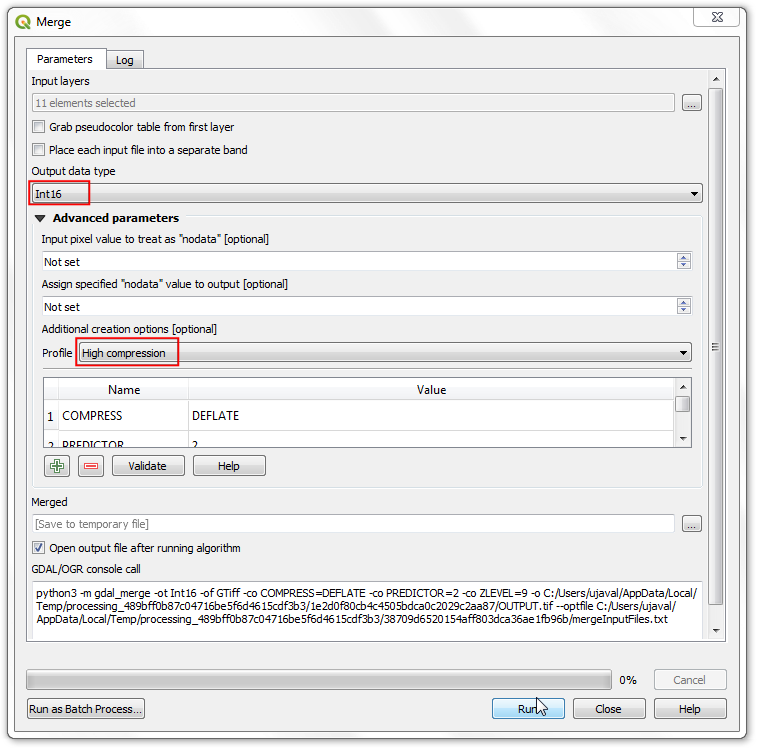
Wir werden jetzt die Eingaben für das Skript festlegen. Die Vorlage enthält die Definition eines
INPUT
Vektorlayers und einesOUTPUT
Layers. Wir werden 2 weitere Eingaben hinzufügen, die es dem Benutzer erlauben, einDISSOLVE_FIELD
und einSUM_FIELD
auszuwählen. Wir fügen zu Beginn des Skripts einen weiteren Import hinzu und fügen den folgenden Programmiercode in derinitAlgorithm
Methode ein. Klicke auf den Knopf Skript ausführen, um eine Vorschau der Änderungen zu sehen.
from qgis.core import QgsProcessingParameterField
self.addParameter(
QgsProcessingParameterField(
self.DISSOLVE_FIELD,
'Choose Dissolve Field',
'',
self.INPUT))
self.addParameter(
QgsProcessingParameterField(
self.SUM_FIELD,
'Choose Sum Field',
'',
self.INPUT))
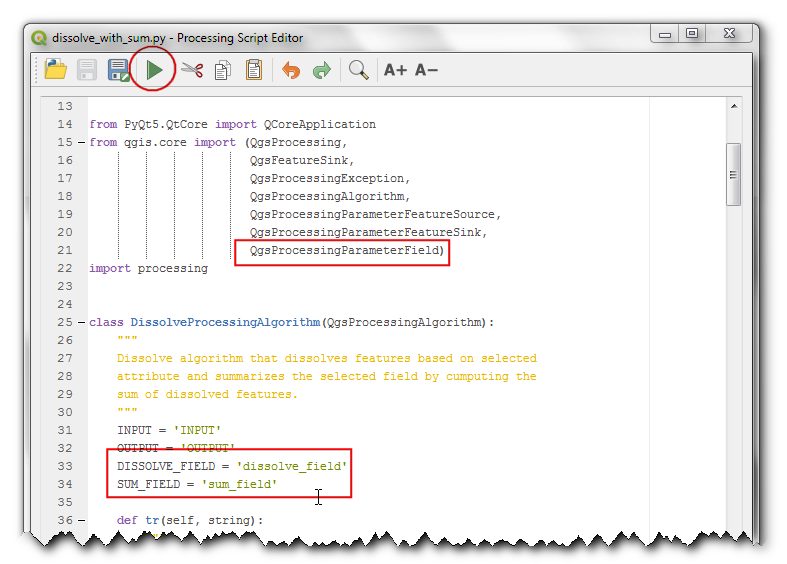
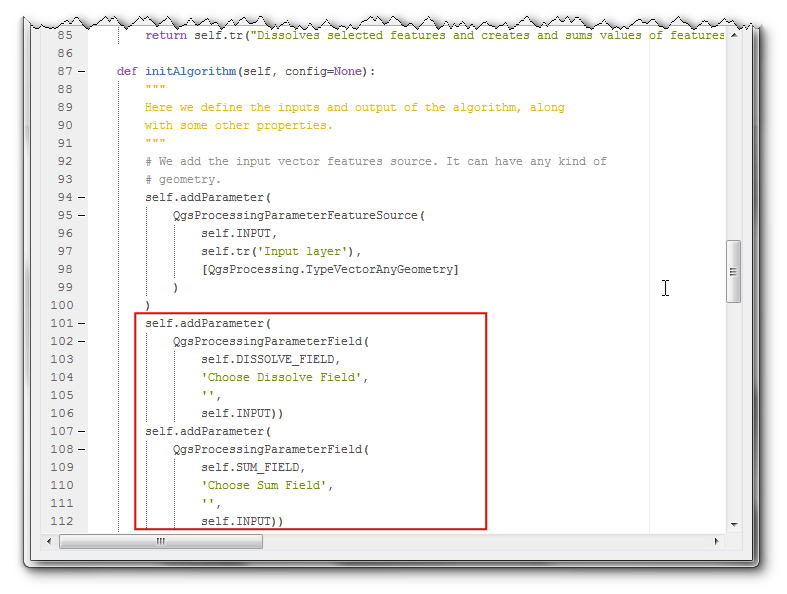
Wir sehen das Dialogfenster Dissolve with Sum mit unseren neu definierten Eingabefeldern. Wähle den Layer
ne_10m_admin_0_countries
als Input layer`. Da die Felder Dissolve Field und Sum Fields auf dem Eingabelayer basieren, werden in den Auswahlfeldern nur die Attribute angezeigt, die im Eingabelayer enthalten sind Klicke auf den Schließen Knopf.
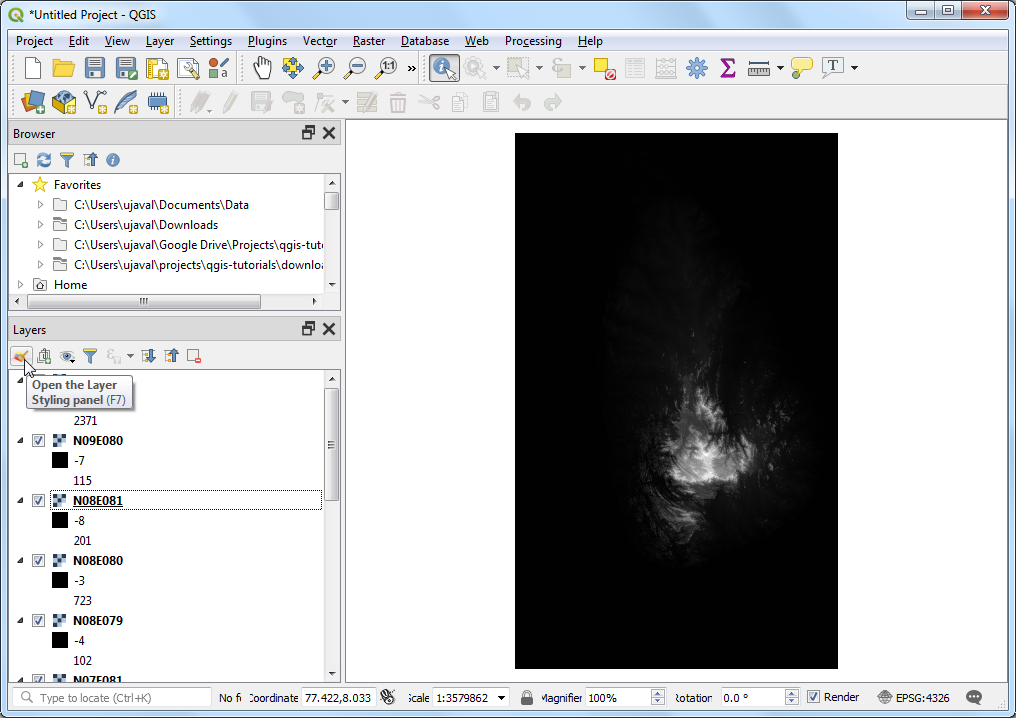
Wir erstellen nun unseren angepassten Programmkode, um die Daten mit der
processAlgorithm
Methode zu verarbeiten. An diese Methode wird einparameters
genanntes Wörterbuch weitergeleitet. Es enthält die Eingaben, die der Benutzer gewählt hat. Es gibt Hilfsmethoden, die es erlauben diese Eingaben zur Erstellung passender Objekte zu verwenden.
from PyQt5.QtCore import QVariant
from qgis.core import QgsField, QgsFields
source = self.parameterAsSource(
parameters,
self.INPUT,
context)
dissolve_field = self.parameterAsString(
parameters,
self.DISSOLVE_FIELD,
context)
sum_field = self.parameterAsString(
parameters,
self.SUM_FIELD,
context)
fields = QgsFields()
fields.append(QgsField(dissolve_field, QVariant.String))
fields.append(QgsField('SUM_' + sum_field, QVariant.Double))
(sink, dest_id) = self.parameterAsSink(
parameters,
self.OUTPUT,
context, fields, source.wkbType(), source.sourceCrs())
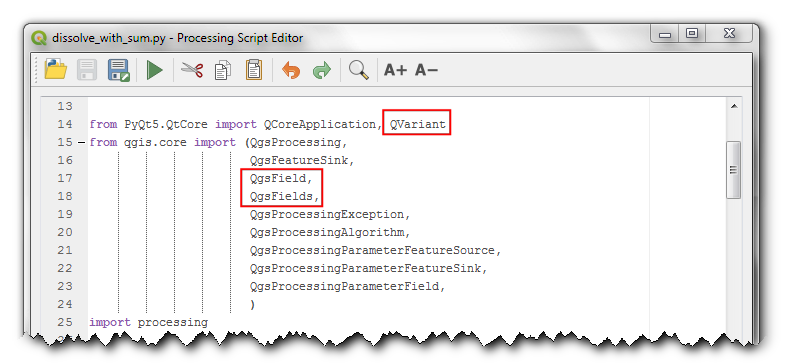
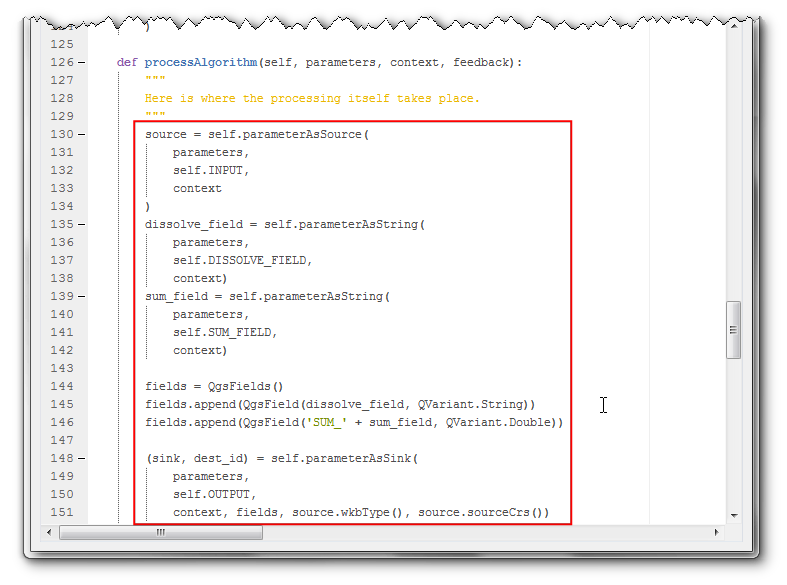
Now we will ready the input features and create a dictionary to hold the unique values from the dissolve_field and the sum of the values from the sum_field. Note the use of
feedback.pushInfo()
method to communicate the status with the user.
feedback.pushInfo('Extracting unique values from dissolve_field and computing sum')
features = source.getFeatures()
unique_values = set(f[dissolve_field] for f in features)
# Get Indices of dissolve field and sum field
dissolveIdx = source.fields().indexFromName(dissolve_field)
sumIdx = source.fields().indexFromName(sum_field)
# Find all unique values for the given dissolve_field and
# sum the corresponding values from the sum_field
sum_unique_values = {}
attrs = [{dissolve_field: f[dissolveIdx], sum_field: f[sumIdx]} for f in source.getFeatures()]
for unique_value in unique_values:
val_list = [ f_attr[sum_field] for f_attr in attrs if f_attr[dissolve_field] == unique_value]
sum_unique_values[unique_value] = sum(val_list)
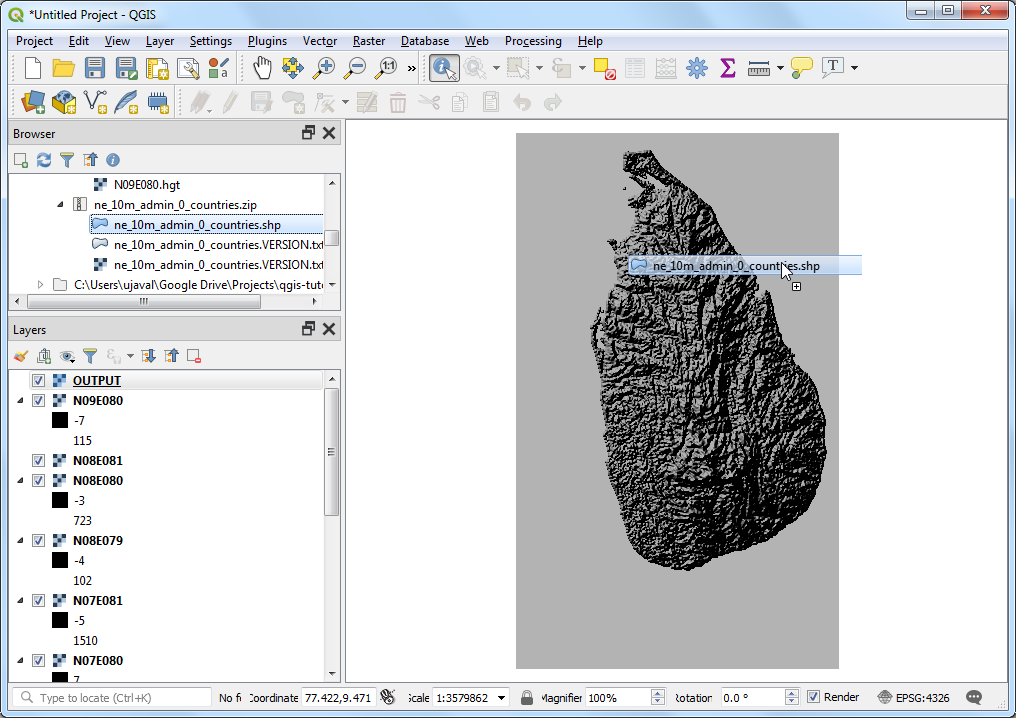
Next, we will call the built-in processing algorithm
native:dissolve
on the input layer to generate the dissolved geometries. Once we have the dissolved geometries, we iterate through the output of the dissolve algorithm and create new features to be added to the output. At the end we return thedest_id
FeatureSink as the output. Now the script is ready. Click the Run button.
Bemerkung
Notice the use of parameters[self.INPUT]
to fetch the input layer from the parameters dictionary directly without defining it as a source. As we are passing the input object to the algorithm without doing anything with it, it’s not necessary to define it as a source.
from qgis.core import QgsFeature
# Running the processing dissolve algorithm
feedback.pushInfo('Dissolving features')
dissolved_layer = processing.run("native:dissolve", {
'INPUT': parameters[self.INPUT],
'FIELD': dissolve_field,
'OUTPUT': 'memory:'
}, context=context, feedback=feedback)['OUTPUT']
# Read the dissolved layer and create output features
for f in dissolved_layer.getFeatures():
new_feature = QgsFeature()
# Set geometry to dissolved geometry
new_feature.setGeometry(f.geometry())
# Set attributes from sum_unique_values dictionary that we had computed
new_feature.setAttributes([f[dissolve_field], sum_unique_values[f[dissolve_field]]])
sink.addFeature(new_feature, QgsFeatureSink.FastInsert)
return {self.OUTPUT: dest_id}
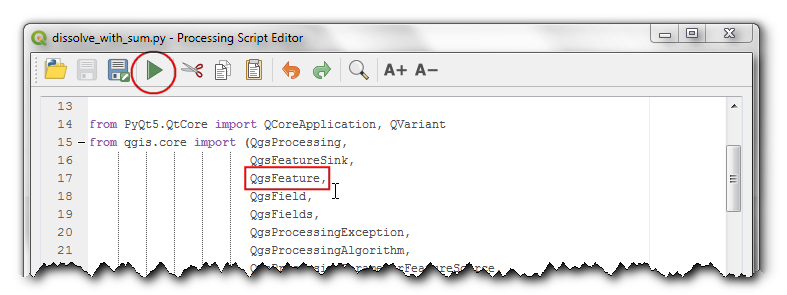
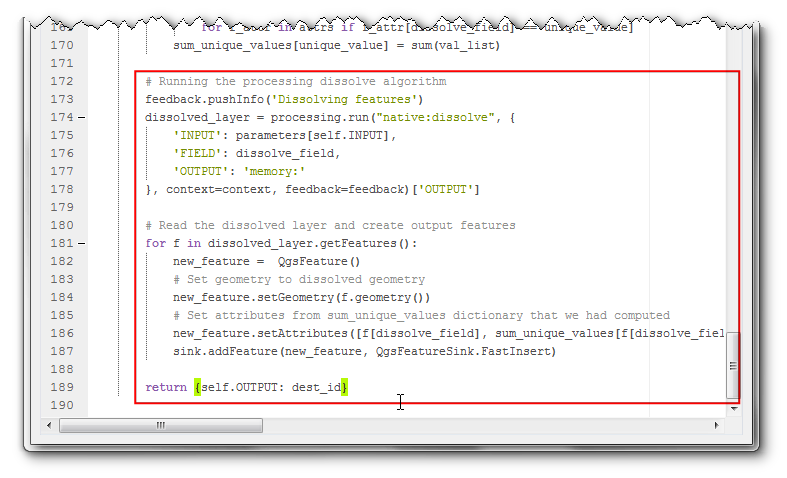
In the Dissolve with Sum, dialog, select
ne_10m_admin_0_countries
as the Input layer,CONTINENT
as the Dissolve field andPOP_EST
as the Sum field. Click Run.
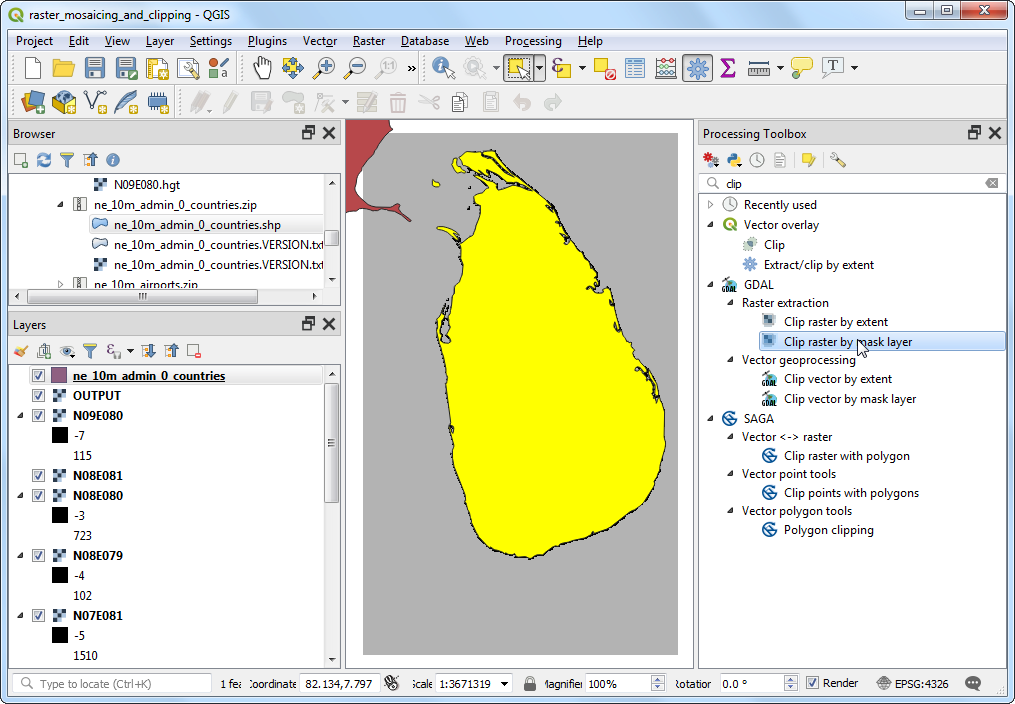
Once the processing is finished, click the Close button and switch to the main QGIS window.
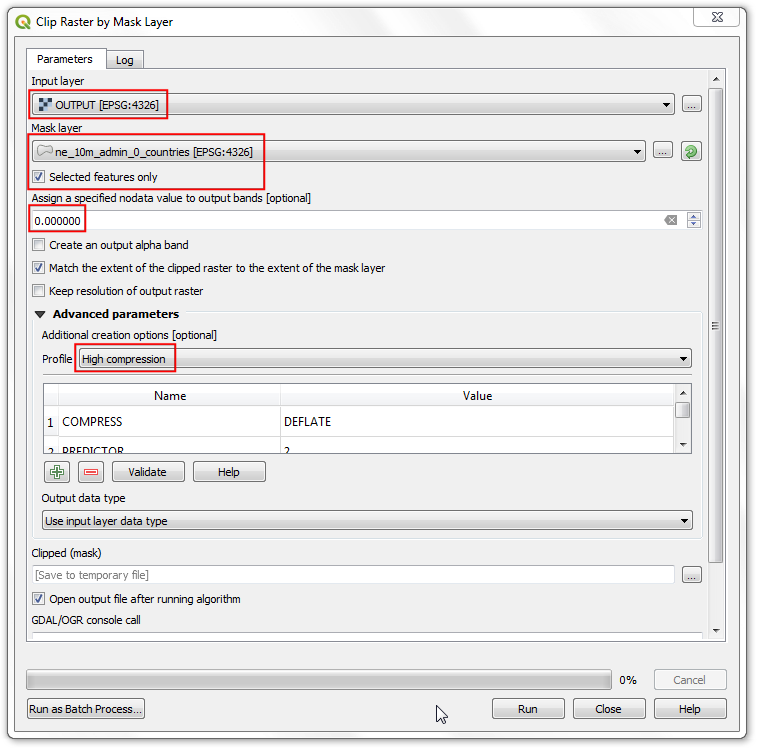
You will see the dissolved output layer with one feature for every continent and the total population summed from the individual countries belonging to that continent.
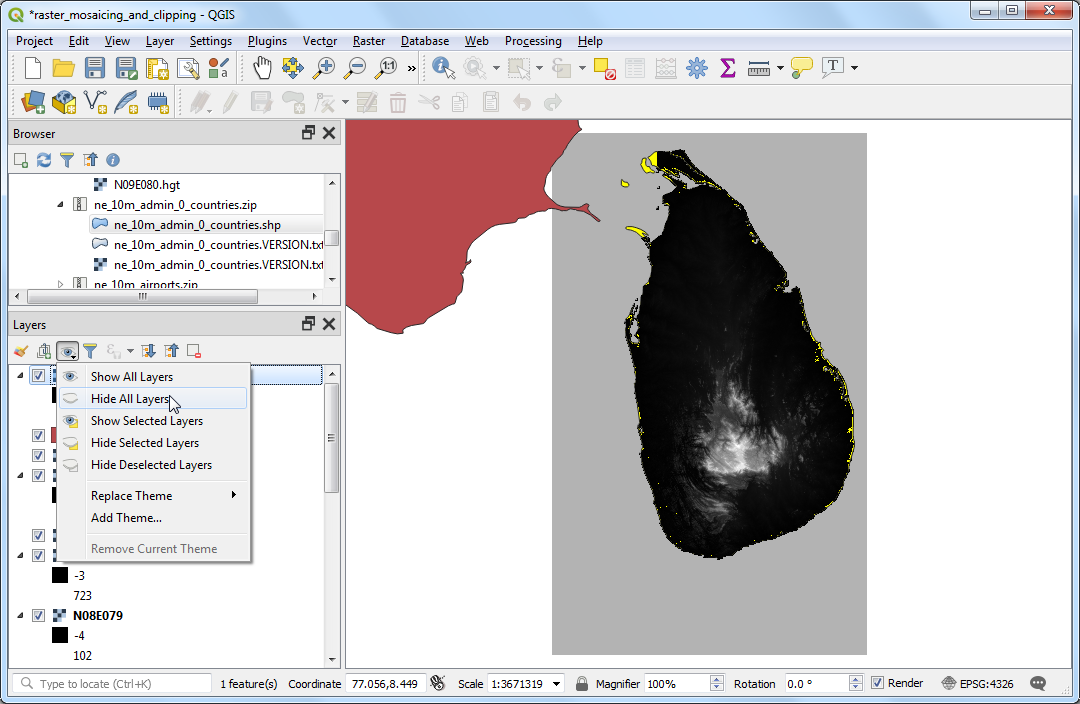
One another advantage of writing processing script is that the methods within the Processing Framework are aware of layer selection and automatically filter your inputs to use only the selected features. This happens because we are defining our input as a
QgsProcessingParameterFeatureSource
. A feature source allows use of ANY object which contains vector features, not just a vector layer, so when there are selected features in your layer and ask Processing to use selected features, the input is passed on to your script as aQgsProcessingFeatureSource
object containing selected features and not the full vector layer. Here’s a quick demonstration of this functionality. Let’s say we want to dissolve only certain continents. Let’s create a selection using Select feature by Expression tool.
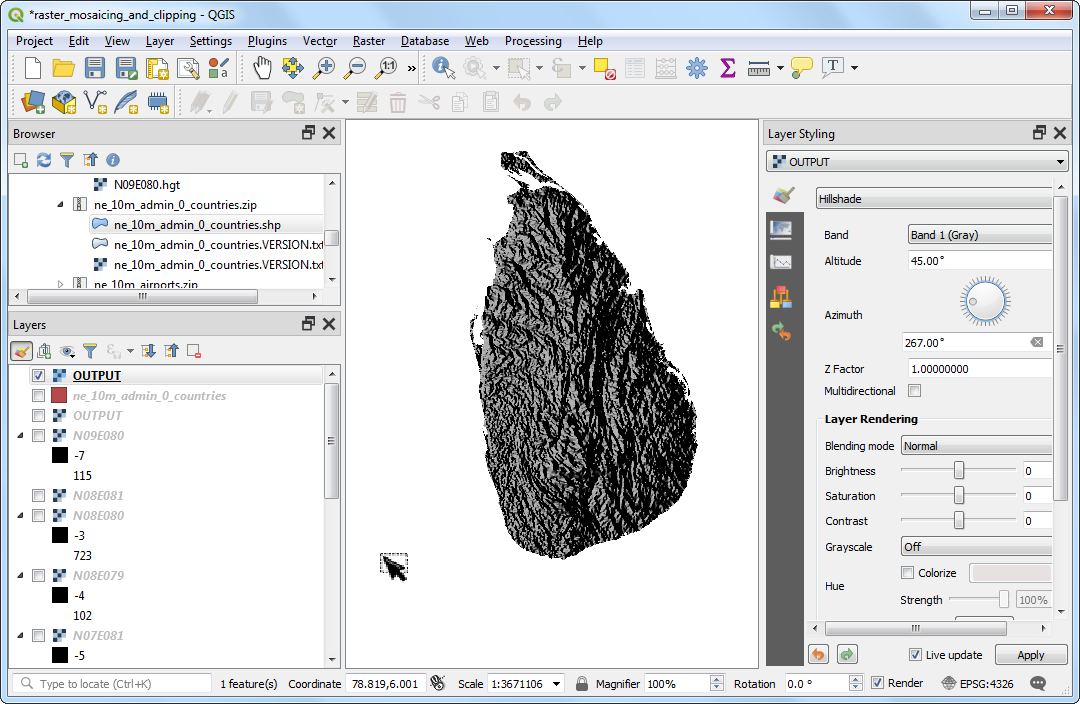
Enter the following expression to select features from North and South America and click Select.
"CONTINENT" = 'North America' OR "CONTINENT" = 'South America'
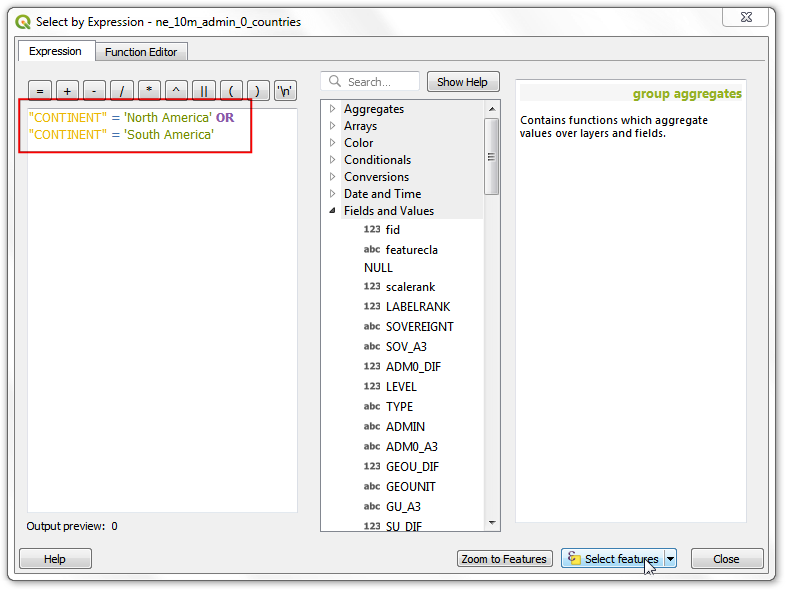
You will see the selected features highlighted in yellow. Locate the
dissolve_with_sum
script and double-click it to run it.
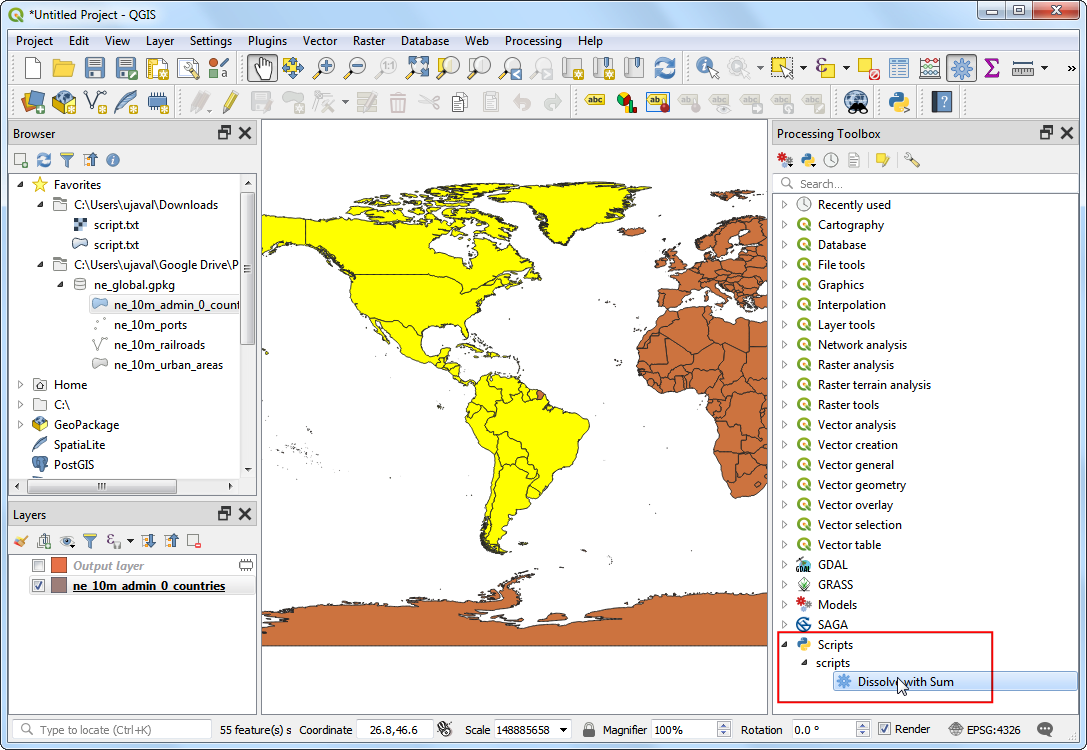
In the Dissolve with Sum dialog, select the
ne_10m_admin_0_countries
as the Input layer. This time, make sure you check the Selected features only box. ChooseSUBREGION
as the Dissolve field andPOP_EST
as the Sum field.
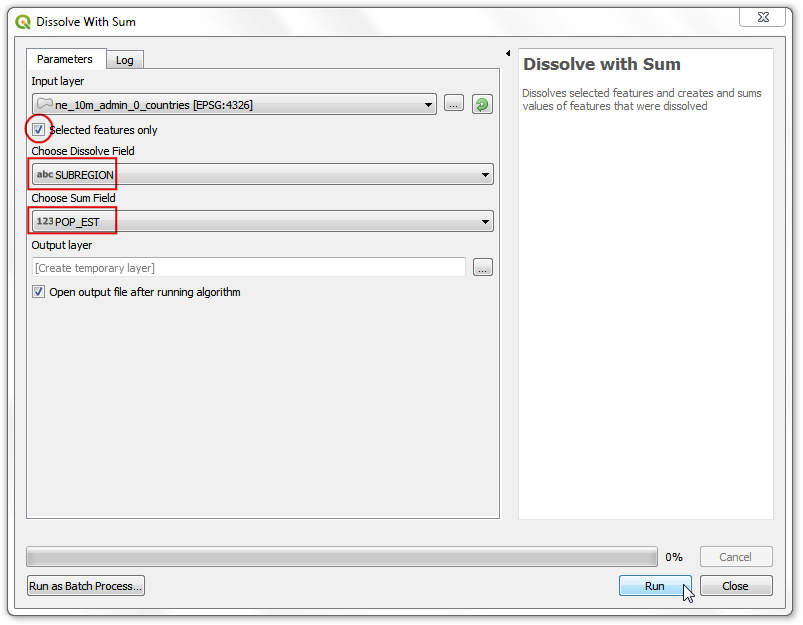
Once the processing finishes, click Close and switch back to the main QGIS window. You will notice a new layer with only the selected features dissolved. Click Identify button and click on a feature to inspect and verify that the script worked correctly.
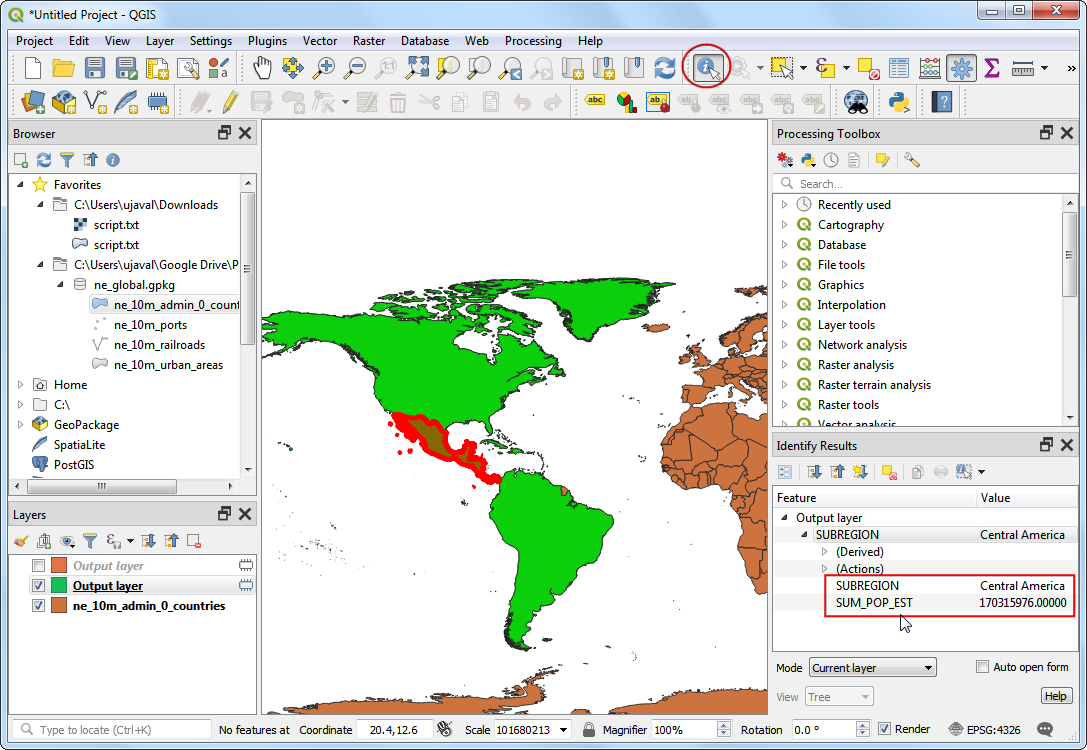
Below is the complete script for reference. You may modify it to suit your needs.
# -*- coding: utf-8 -*-
"""
***************************************************************************
* *
* This program is free software; you can redistribute it and/or modify *
* it under the terms of the GNU General Public License as published by *
* the Free Software Foundation; either version 2 of the License, or *
* (at your option) any later version. *
* *
***************************************************************************
"""
from PyQt5.QtCore import QCoreApplication, QVariant
from qgis.core import (QgsProcessing,
QgsFeatureSink,
QgsFeature,
QgsField,
QgsFields,
QgsProcessingException,
QgsProcessingAlgorithm,
QgsProcessingParameterFeatureSource,
QgsProcessingParameterFeatureSink,
QgsProcessingParameterField,
)
import processing
class DissolveProcessingAlgorithm(QgsProcessingAlgorithm):
"""
Dissolve algorithm that dissolves features based on selected
attribute and summarizes the selected field by cumputing the
sum of dissolved features.
"""
INPUT = 'INPUT'
OUTPUT = 'OUTPUT'
DISSOLVE_FIELD = 'dissolve_field'
SUM_FIELD = 'sum_field'
def tr(self, string):
"""
Returns a translatable string with the self.tr() function.
"""
return QCoreApplication.translate('Processing', string)
def createInstance(self):
return DissolveProcessingAlgorithm()
def name(self):
"""
Returns the algorithm name, used for identifying the algorithm. This
string should be fixed for the algorithm, and must not be localised.
The name should be unique within each provider. Names should contain
lowercase alphanumeric characters only and no spaces or other
formatting characters.
"""
return 'dissolve_with_sum'
def displayName(self):
"""
Returns the translated algorithm name, which should be used for any
user-visible display of the algorithm name.
"""
return self.tr('Dissolve with Sum')
def group(self):
"""
Returns the name of the group this algorithm belongs to. This string
should be localised.
"""
return self.tr('scripts')
def groupId(self):
"""
Returns the unique ID of the group this algorithm belongs to. This
string should be fixed for the algorithm, and must not be localised.
The group id should be unique within each provider. Group id should
contain lowercase alphanumeric characters only and no spaces or other
formatting characters.
"""
return 'scripts'
def shortHelpString(self):
"""
Returns a localised short helper string for the algorithm. This string
should provide a basic description about what the algorithm does and the
parameters and outputs associated with it..
"""
return self.tr("Dissolves selected features and creates and sums values of features that were dissolved")
def initAlgorithm(self, config=None):
"""
Here we define the inputs and output of the algorithm, along
with some other properties.
"""
# We add the input vector features source. It can have any kind of
# geometry.
self.addParameter(
QgsProcessingParameterFeatureSource(
self.INPUT,
self.tr('Input layer'),
[QgsProcessing.TypeVectorAnyGeometry]
)
)
self.addParameter(
QgsProcessingParameterField(
self.DISSOLVE_FIELD,
'Choose Dissolve Field',
'',
self.INPUT))
self.addParameter(
QgsProcessingParameterField(
self.SUM_FIELD,
'Choose Sum Field',
'',
self.INPUT))
# We add a feature sink in which to store our processed features (this
# usually takes the form of a newly created vector layer when the
# algorithm is run in QGIS).
self.addParameter(
QgsProcessingParameterFeatureSink(
self.OUTPUT,
self.tr('Output layer')
)
)
def processAlgorithm(self, parameters, context, feedback):
"""
Here is where the processing itself takes place.
"""
source = self.parameterAsSource(
parameters,
self.INPUT,
context
)
dissolve_field = self.parameterAsString(
parameters,
self.DISSOLVE_FIELD,
context)
sum_field = self.parameterAsString(
parameters,
self.SUM_FIELD,
context)
fields = QgsFields()
fields.append(QgsField(dissolve_field, QVariant.String))
fields.append(QgsField('SUM_' + sum_field, QVariant.Double))
(sink, dest_id) = self.parameterAsSink(
parameters,
self.OUTPUT,
context, fields, source.wkbType(), source.sourceCrs())
# Create a dictionary to hold the unique values from the
# dissolve_field and the sum of the values from the sum_field
feedback.pushInfo('Extracting unique values from dissolve_field and computing sum')
features = source.getFeatures()
unique_values = set(f[dissolve_field] for f in features)
# Get Indices of dissolve field and sum field
dissolveIdx = source.fields().indexFromName(dissolve_field)
sumIdx = source.fields().indexFromName(sum_field)
# Find all unique values for the given dissolve_field and
# sum the corresponding values from the sum_field
sum_unique_values = {}
attrs = [{dissolve_field: f[dissolveIdx], sum_field: f[sumIdx]}
for f in source.getFeatures()]
for unique_value in unique_values:
val_list = [ f_attr[sum_field]
for f_attr in attrs if f_attr[dissolve_field] == unique_value]
sum_unique_values[unique_value] = sum(val_list)
# Running the processing dissolve algorithm
feedback.pushInfo('Dissolving features')
dissolved_layer = processing.run("native:dissolve", {
'INPUT': parameters[self.INPUT],
'FIELD': dissolve_field,
'OUTPUT': 'memory:'
}, context=context, feedback=feedback)['OUTPUT']
# Read the dissolved layer and create output features
for f in dissolved_layer.getFeatures():
new_feature = QgsFeature()
# Set geometry to dissolved geometry
new_feature.setGeometry(f.geometry())
# Set attributes from sum_unique_values dictionary that we had computed
new_feature.setAttributes([f[dissolve_field], sum_unique_values[f[dissolve_field]]])
sink.addFeature(new_feature, QgsFeatureSink.FastInsert)
return {self.OUTPUT: dest_id}
If you want to give feedback or share your experience with this tutorial, please comment below. (requires GitHub account)